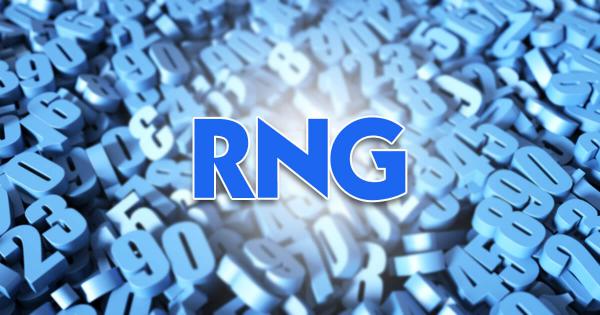
Definition
Random number generations known as 'RNG' is widely used in different fields from games to apps to websites, to make things unpredictable and natural, in competitive programming RNG is used to create random test data or to solve problems with high probability.
How generate random numbers
To generate random numbers we usually need to choose a 'seed' (a seed is a base value used to calculate the random values, hence RNG is not really random) in most cases we use the current time as a seed since it is unpredictable, then we can use the random function to get a value in the range \( [0,1) \), now we need to figure out how to generate a value between \( x and y inclusive \).
Note: 1 is not in the range of the random function ( \( [0,1) \) ) so it will never return 1.
Let's look at the simpler version first. the range \( [0, x) \), we can see that if we multiply a rangw by some value the range size will change, \( [0,1) * 5 \) we will get \( [0,5)\) so to get \( [0, x) \) we will multiply the range by \( x\).
Now for the general case, the range \( [x, y) \), first we will change the size of the range to \( (length = y - x + 1) \) and we get \( [0, 1) * length = [0, length) = [0,y - x + 1) \), now to fix the left boundary we simply shift the range by \( x \), \( [0, y - x + 1) + x = [0 + x, (y - x + 1) + x) = [x , y + 1) \).
Note: that the \( y+1 \) is not in the range of the random function.
Steps to generate random values
Let's summarize the steps we used to generate a random integer between \(x and y inclusive\):
- Set the seed of the random function (current time is usually used).
- Multiply the random function range by the size of the needed range ( \( random() * (y -x + 1) \) ).
- Shift the random function range by the left boundary ( ( \( (random() * (y -x + 1)) + x \) ).
- Convert the result from float to integer.
RNG Implementation
Python Implementation
from random import seed
from random import random
import random
x = 3
y = 10
Length = y - x + 1
for _ in range(0,10):
currentValuue = random.random() * Length
currentValuue = currentValuue + x
currentValuue = int(currentValuue)
print(currentValuue, end=' ')
Output:
5 4 4 3 7 8 4 10 9 7
C++ Implementation
#include <iostream>
#include <random>
#include <time.h>
using namespace std;
int main(){
srand(time(0));
int x = 3,y = 10;
int length = y - x + 1;
for(int i=1;i<=10;i++){
int currentValue = rand() % length;
currentValue = currentValue + x;
cout << currentValue << ' ';
}
}
Output:
10 8 5 4 5 7 10 3 9 7